The SystemUIOverlayWindow
management system provides a way for you to display and
manage views in the SystemUIOverlayWindow
. Currently, this window is used for views
including the full screen user switcher, notification panel, and keyguard. This page doesn't:
- Create restrictions around what the OEM can add to the window.
- Force you to adopt the abstractions described on this page.
Overview
You can use the SystemUIOverlayWindow
Management System to show views such as the
legal notice, fullscreen user switcher, rear view camera, HVAC controls, and keyguard. This
window lies outside the app space and gives you control over the view's Z-ordering,
reveal/conceal triggers, and overall customizations including view placement, size, transparency,
and color. At the same time, you needn't be concerned with the state of system bars or other
System UI objects that need to be hidden or shown when their respective view is hidden or shown.
To take advantage of SystemUIOverlayWindow
, you create view controllers for you view
mediators. The mediators are passed to the window's global state controller. These view mediators:
- Coordinate between view controllers.
- House business logic for view controllers.
View controllers (coordinated by view mediators):
- Own its view.
- Create setters through which
OverlayViewsMediator
can attach business logic. - Create their view's reveal and conceal animations.
SystemUIOverlayWindow
Manager, a SystemUI component,serves as an entry point to
initialize and register mediators with the global state controller while the global state controller
ties into the view controllers in such a way that mediators can directly call the view controllers
to show and hide views in the window.
OverlayViewController
OverlayViewController
is responsible for the view displayed in SystemUIOverlayWindow
and controls how the view is
revealed and hidden. It also enables required listeners to be attached so that it can be tied
to business logic.
Important method signatures
/**
* Owns a {@link View} that is present in SystemUIOverlayWindow
.
*/
public class OverlayViewController {
/**
* Shows content of {@link OverlayViewController}.
*
* Should be used to show view externally and in particular by {@link OverlayViewMediator}.
*/
public final void start();
/**
* Hides content of {@link OverlayViewController}.
*
* Should be used to hide view externally and in particular by {@link OverlayViewMediator}.
*/
public final void stop();
/**
* Inflate layout owned by controller.
*/
public final void inflate(ViewGroup baseLayout);
/**
* Called once inflate finishes.
*/
protected void onFinishInflate();
/**
* Returns {@code true} if layout owned by controller has been inflated.
*/
public final boolean isInflated();
/**
* Subclasses should override this method to implement reveal animations and implement logic
* specific to when the layout owned by the controller is shown.
*
* Should only be overridden by Superclass but not called by any {@link OverlayViewMediator}.
*/
protected void showInternal();
/**
* Subclasses should override this method to implement conceal animations and implement logic
* specific to when the layout owned by the controller is hidden.
*
* Should only be overridden by Superclass but not called by any {@link OverlayViewMediator}.
*/
protected void hideInternal();
/**
* Provides access to layout owned by controller.
*/
protected final View getLayout();
/** Returns the {@link OverlayViewGlobalStateController}. */
protected final OverlayViewGlobalStateController getOverlayViewGlobalStateController();
/** Returns whether the view controlled by this controller is visible. */
public final boolean isVisible();
/**
* Returns the ID of the focus area that should receive focus when this view is the
* topmost view or {@link View#NO_ID} if there is no focus area.
*/
@IdRes
protected int getFocusAreaViewId();
/** Returns whether the view controlled by this controller has rotary focus. */
protected final boolean hasRotaryFocus();
/**
* Sets whether this view allows rotary focus. This should be set to {@code true} for the
* topmost layer in the overlay window and {@code false} for the others.
*/
public void setAllowRotaryFocus(boolean allowRotaryFocus);
/**
* Refreshes the rotary focus in this view if we are in rotary mode. If the view already has
* rotary focus, it leaves the focus alone. Returns {@code true} if a new view was focused.
*/
public boolean refreshRotaryFocusIfNeeded();
/**
* Returns {@code true} if heads up notifications should be displayed over this view.
*/
protected boolean shouldShowHUN();
/**
* Returns {@code true} if navigation bar insets should be displayed over this view. Has no
* effect if {@link #shouldFocusWindow} returns {@code false}.
*/
protected boolean shouldShowNavigationBarInsets();
/**
* Returns {@code true} if status bar insets should be displayed over this view. Has no
* effect if {@link #shouldFocusWindow} returns {@code false}.
*/
protected boolean shouldShowStatusBarInsets();
/**
* Returns {@code true} if this view should be hidden during the occluded state.
*/
protected boolean shouldShowWhenOccluded();
/**
* Returns {@code true} if the window should be focued when this view is visible. Note that
* returning {@code false} here means that {@link #shouldShowStatusBarInsets} and
* {@link #shouldShowNavigationBarInsets} will have no effect.
*/
protected boolean shouldFocusWindow();
/**
* Returns {@code true} if the window should use stable insets. Using stable insets means that
* even when system bars are temporarily not visible, inset from the system bars will still be
* applied.
*
* NOTE: When system bars are hidden in transient mode, insets from them will not be applied
* even when the system bars become visible. Setting the return value to {@true} here can
* prevent the OverlayView from overlapping with the system bars when that happens.
*/
protected boolean shouldUseStableInsets();
/**
* Returns the insets types to fit to the sysui overlay window when this
* {@link OverlayViewController} is in the foreground.
*/
@WindowInsets.Type.InsetsType
protected int getInsetTypesToFit();
/**
* Optionally returns the sides of enabled system bar insets to fit to the sysui overlay window
* when this {@link OverlayViewController} is in the foreground.
*
* For example, if the bottom and left system bars are enabled and this method returns
* WindowInsets.Side.LEFT, then the inset from the bottom system bar will be ignored.
*
* NOTE: By default, this method returns {@link #INVALID_INSET_SIDE}, so insets to fit are
* defined by {@link #getInsetTypesToFit()}, and not by this method, unless it is overridden
* by subclasses.
*
* NOTE: {@link #NO_INSET_SIDE} signifies no insets from any system bars will be honored. Each
* {@link OverlayViewController} can first take this value and add sides of the system bar
* insets to honor to it.
*
* NOTE: If getInsetSidesToFit is overridden to return {@link WindowInsets.Side}, it always
* takes precedence over {@link #getInsetTypesToFit()}. That is, the return value of {@link
* #getInsetTypesToFit()} will be ignored.
*/
@WindowInsets.Side.InsetsSide
protected int getInsetSidesToFit();
}
OverlayPanelViewController
OverlayPanelViewController
controller extends OverlayViewController
and provides additional dragging animation capabilities to its superclass.
OverlayViewMediator
OverlayViewMediator
houses business logic that reveals or hides
multiple OverlayViewController
instances, so, in a way it also manages the
coordination between view controllers.
/** * Controls when to show and hide {@link OverlayViewController}(s). */ public interface OverlayViewMediator { /** * Register listeners that could use ContentVisibilityAdjuster to show/hide content. * * Note that we do not unregister listeners because SystemUI components are expected to live * for the lifecycle of the device. */ void registerListeners(); /** * Allows for post-inflation callbacks and listeners to be set inside required {@link * OverlayViewController}(s). */ void setupOverlayContentViewControllers(); }
SystemUIOverlayWindowManager
SystemUIOverlayWindowManager
is responsible for being the SystemUI object that serves an entry point for the
SystemUIOverlayWindow
Management System to initialize and register
OverlayViewMediator
instances with OverlayViewGlobalStateController
.
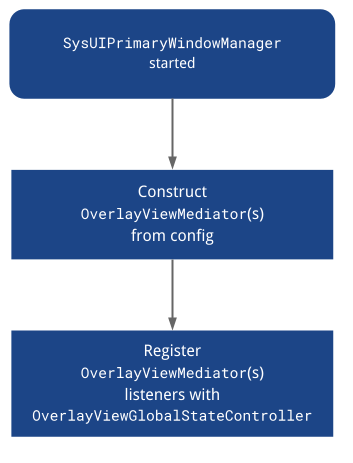
OverlayViewGlobalStateController
OverlayViewGlobalStateController
receives calls from
OverlayViewController
instances to reveal or conceal themselves. Therefore, it also
holds the state of what is shown or hidden in SystemUIOverlayWindow
.
The show view flow is illustrated below:
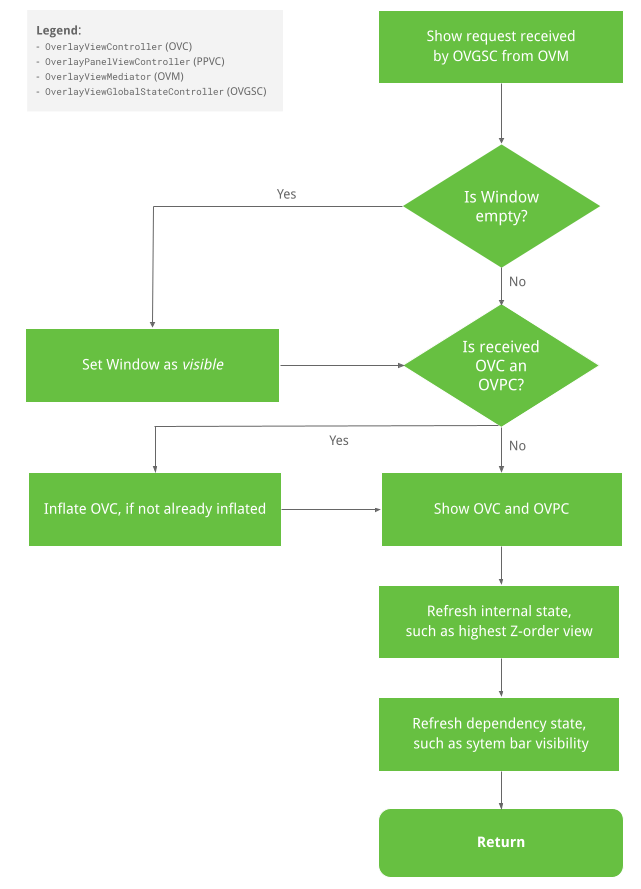
Hide view flow
The hide view flow is illustrated below:
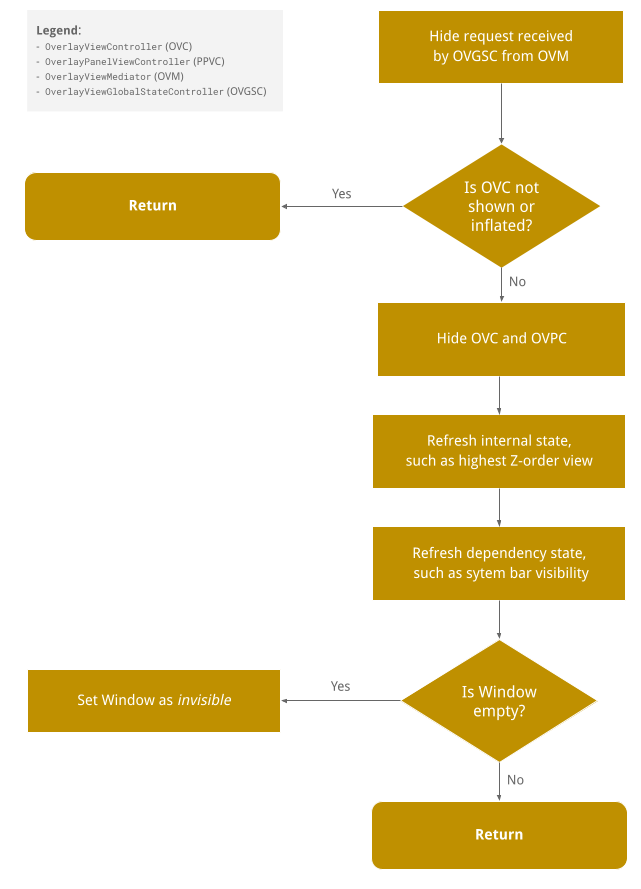
Public method signatures
Public method signatures are encoded as follows:
/**
* This controller is responsible for the following:
* <p><ul>
* <li>Holds the global state for SystemUIOverlayWindow.
* <li>Allows {@link SystemUIOverlayWindowManager} to register {@link OverlayViewMediator}(s).
* <li>Enables {@link OverlayViewController)(s) to reveal/conceal themselves while respecting the
* global state of SystemUIOverlayWindow.
* </ul>
*/
@SysUISingleton
public class OverlayViewGlobalStateController {
/**
* Register {@link OverlayViewMediator} to use in SystemUIOverlayWindow
.
*/
public void registerMediator(OverlayViewMediator overlayViewMediator);
/**
* Show content in Overlay Window using {@link OverlayPanelViewController}.
*
* This calls {@link OverlayViewGlobalStateController#showView(OverlayViewController, Runnable)}
* where the runnable is nullified since the actual showing of the panel is handled by the
* controller itself.
*/
public void showView(OverlayPanelViewController panelViewController);
/**
* Show content in Overlay Window using {@link OverlayViewController}.
*/
public void showView(OverlayViewController viewController, @Nullable Runnable show);
/**
* Hide content in Overlay Window using {@link OverlayPanelViewController}.
*
* This calls {@link OverlayViewGlobalStateController#hideView(OverlayViewController, Runnable)}
* where the runnable is nullified since the actual hiding of the panel is handled by the
* controller itself.
*/
public void hideView(OverlayPanelViewController panelViewController);
/**
* Hide content in Overlay Window using {@link OverlayViewController}.
*/
public void hideView(OverlayViewController viewController, @Nullable Runnable hide);
/** Returns {@code true} is the window is visible. */
public boolean isWindowVisible();
/**
* Sets the {@link android.view.WindowManager.LayoutParams#FLAG_ALT_FOCUSABLE_IM} flag of the
* sysui overlay window.
*/
public void setWindowNeedsInput(boolean needsInput);
/** Returns {@code true} if the window is focusable. */
public boolean isWindowFocusable();
/** Sets the focusable flag of the sysui overlawy window. */
public void setWindowFocusable(boolean focusable);
/** Inflates the view controlled by the given view controller. */
public void inflateView(OverlayViewController viewController);
/**
* Return {@code true} if OverlayWindow is in a state where HUNs should be displayed above it.
*/
public boolean shouldShowHUN();
/**
* Set the OverlayViewWindow to be in occluded or unoccluded state. When OverlayViewWindow is
* occluded, all views mounted to it that are not configured to be shown during occlusion will
* be hidden.
*/
public void setOccluded(boolean occluded);
}
How to add a view to SysUIOverlayWindow
For details, see the Codelab.
Step 1: Add a ViewStub to SysUIOverlayWindow
Add ViewStub
to the
window layout.
Step 2: Create an OverlayViewController
Use the new ViewStub
to create a new injectable
OverlayViewController
.
Step 3: OverlayViewMediator
Create a new injectable
OverlayViewMediator
or use an existing one (skip Step 4) and register listeners to hide or show the new
OverlayViewController
.
Step 4: Configure the new OverlayViewMediator
Add your new OverlayViewMediator
to
OverlayWindowModule
and to
config_carSystemUIOverlayViewsMediator
.
Caveats
When SysUIPrimaryWindow
covers the entire screen, any elements under the window
don't register touch events. Therefore, when the window covers the entire screen but its contents
leave some negative space, you can choose to blur out the negative space and attach listeners to
that space to dismiss content in the window.