On devices with a fingerprint sensor, users can enroll one or more fingerprints and use those fingerprints to unlock the device and perform other tasks. Android uses the Fingerprint Hardware Interface Definition Language (HIDL) to connect to a vendor-specific library and fingerprint hardware (for example, a fingerprint sensor).
To implement the Fingerprint HIDL, you must implement IBiometricsFingerprint.hal
in a vendor-specific library.
Fingerprint matching
The fingerprint sensor of a device is generally idle. However, in response to
a call to authenticate
or enroll
, the
fingerprint sensor listens for a touch (the screen might also wake when a user
touches the fingerprint sensor). The high-level flow of fingerprint matching
includes the following steps:
- User places a finger on the fingerprint sensor.
- The vendor-specific library determines if there is a fingerprint match in the current set of enrolled fingerprint templates.
- Matching results are passed to
FingerprintService
.
This flow assumes that a fingerprint has already been enrolled on the device, that is, the vendor-specific library has enrolled a template for the fingerprint. For more details, see Authentication.
Architecture
The Fingerprint HAL interacts with the following components.
BiometricManager
interacts directly with an app in an app process. Each app has an instance ofIBiometricsFingerprint.hal
FingerprintService
operates in the system process, which handles communication with fingerprint HAL.- Fingerprint HAL is a C/C++ implementation of the IBiometricsFingerprint HIDL interface. This contains the vendor-specific library that communicates with the device-specific hardware.
- Keystore API and KeyMint (previously Keymaster) components provide hardware-backed cryptography for secure key storage in a secure environment, such as the Trusted Execution Environment (TEE).
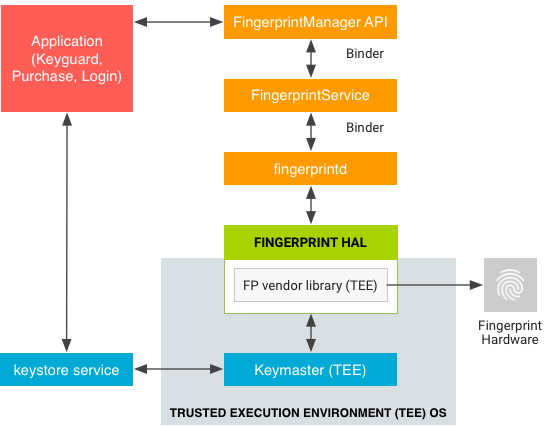
A vendor-specific HAL implementation must use the communication protocol required by a TEE. Raw images and processed fingerprint features must not be passed in untrusted memory. All such biometric data needs to be stored in the secure hardware such as the TEE. Rooting must not be able to compromise biometric data.
FingerprintService
and fingerprintd
make calls through the Fingerprint HAL to
the vendor-specific library to enroll fingerprints and perform other
operations.
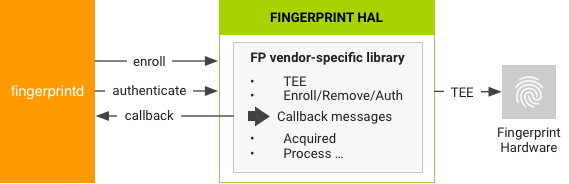
Implementation guidelines
The following Fingerprint HAL guidelines are designed to ensure that fingerprint data is not leaked and is removed when a user is removed from a device:
- Raw fingerprint data or derivatives (for example, templates) must never be accessible from outside the sensor driver or TEE. If the hardware supports a TEE, hardware access must be limited to the TEE and protected by an SELinux policy. The Serial Peripheral Interface (SPI) channel must be accessible only to the TEE and there must be an explicit SELinux policy on all device files.
- Fingerprint acquisition, enrollment, and recognition must occur inside the TEE.
- Only the encrypted form of the fingerprint data can be stored on the file system, even if the file system itself is encrypted.
- Fingerprint templates must be signed with a private, device-specific key. For Advanced Encryption Standard (AES), at a minimum a template must be signed with the absolute file-system path, group, and finger ID such that template files are inoperable on another device or for anyone other than the user that enrolled them on the same device. For example, copying fingerprint data from a different user on the same device or from another device must not work.
- Implementations must either use the file-system path provided by the
setActiveGroup()
function or provide a way to erase all user template data when the user is removed. It's strongly recommended that fingerprint template files be stored as encrypted and stored in the path provided. If this is infeasible due to TEE storage requirements, the implementer must add hooks to ensure removal of the data when the user is removed.
Fingerprint methods
The Fingerprint HIDL interface contains the following major methods in
IBiometricsFingerprint.hal
.
Method | Description |
---|---|
enroll() |
Switches the HAL state machine to start the collection and storage of a fingerprint template. When enrollment is complete, or after a timeout, the HAL state machine returns to the idle state. |
preEnroll() |
Generates a unique token to indicate the start of a
fingerprint enrollment. Provides a token to the enroll function to
ensure there was prior authentication, for example, using a password. To prevent
tampering, the token is wrapped after the device
credential is confirmed. The token must be checked during enrollment to verify
that it's still valid. |
getAuthenticatorId() |
Returns a token associated with the current fingerprint set. |
cancel() |
Cancels pending enroll or authenticate operations. The HAL state machine is returned to the idle state. |
enumerate() |
Synchronous call for enumerating all known fingerprint templates. |
remove() |
Deletes a fingerprint template. |
setActiveGroup() |
Restricts a HAL operation to a set of fingerprints that belong to a specified group, identified by a group identifier (GID). |
authenticate() |
Authenticates a fingerprint-related operation (identified by an operation ID). |
setNotify() |
Registers a user function that receives notifications from the HAL. If the HAL state machine is in a busy state, the function is blocked until the HAL leaves the busy state. |
postEnroll() |
Finishes the enroll operation and invalidates the
preEnroll() generated challenge. This must be called at the end of a
multifinger enrollment session to indicate that no more fingers may be added. |
For more details on these, refer to the comments in IBiometricsFingerprint.hal
.