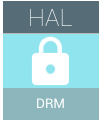
This document provides an overview of the Android digital rights management (DRM) framework and introduces the interfaces that a DRM plugin must implement. This document doesn't describe robustness rules or compliance rules that may be defined by a DRM scheme.
Framework
The Android platform provides an extensible DRM framework that lets apps manage rights-protected content according to the license constraints associated with the content. The DRM framework supports many DRM schemes; which DRM schemes a device supports is up to the device manufacturer. The DRM framework provides a unified interface for application developers and hides the complexity of DRM operations. The DRM framework provides a consistent operation mode for protected and nonprotected content. DRM schemes can define complex usage models by license metadata. The DRM framework provides the association between DRM content and license, and handles the rights management. This enables the media player to be abstracted from DRM-protected or nonprotected content. See MediaDrm for the class to obtain keys for decrypting protected media streams.
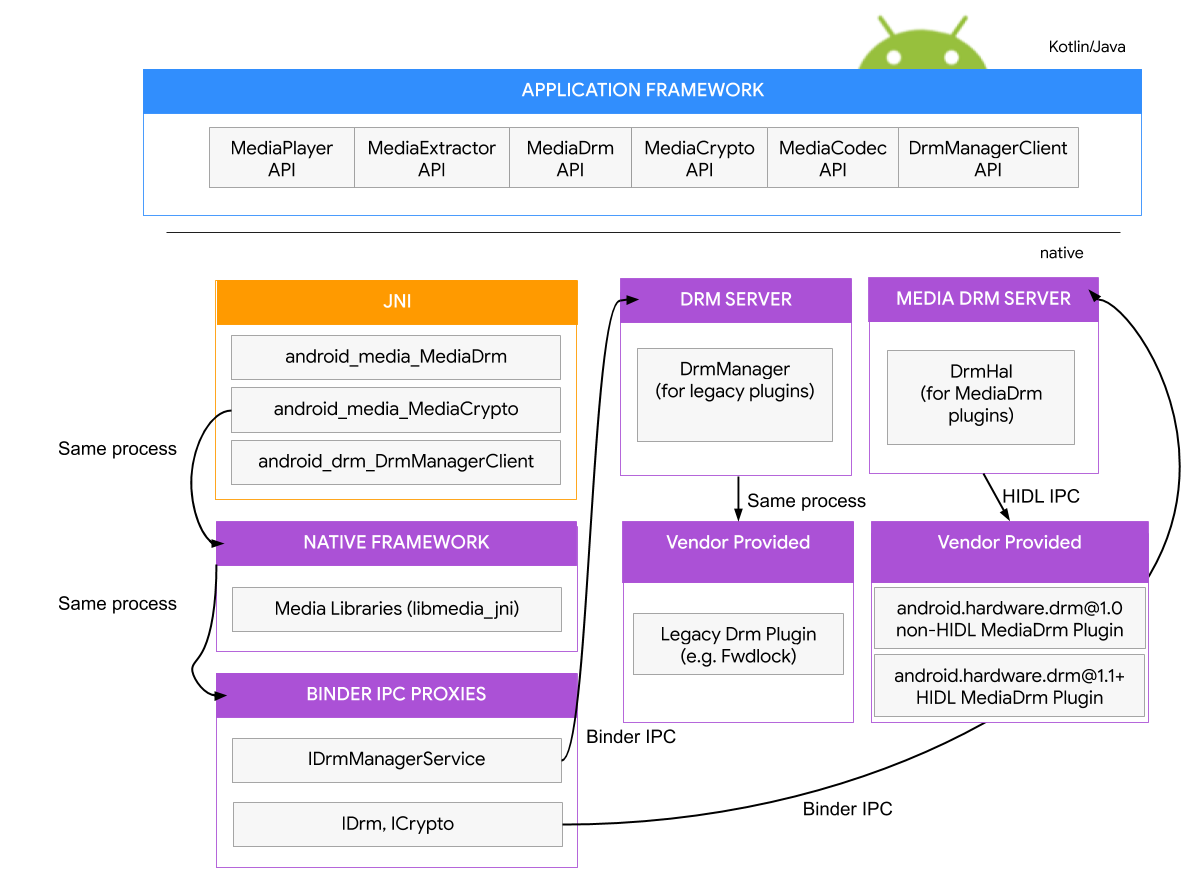
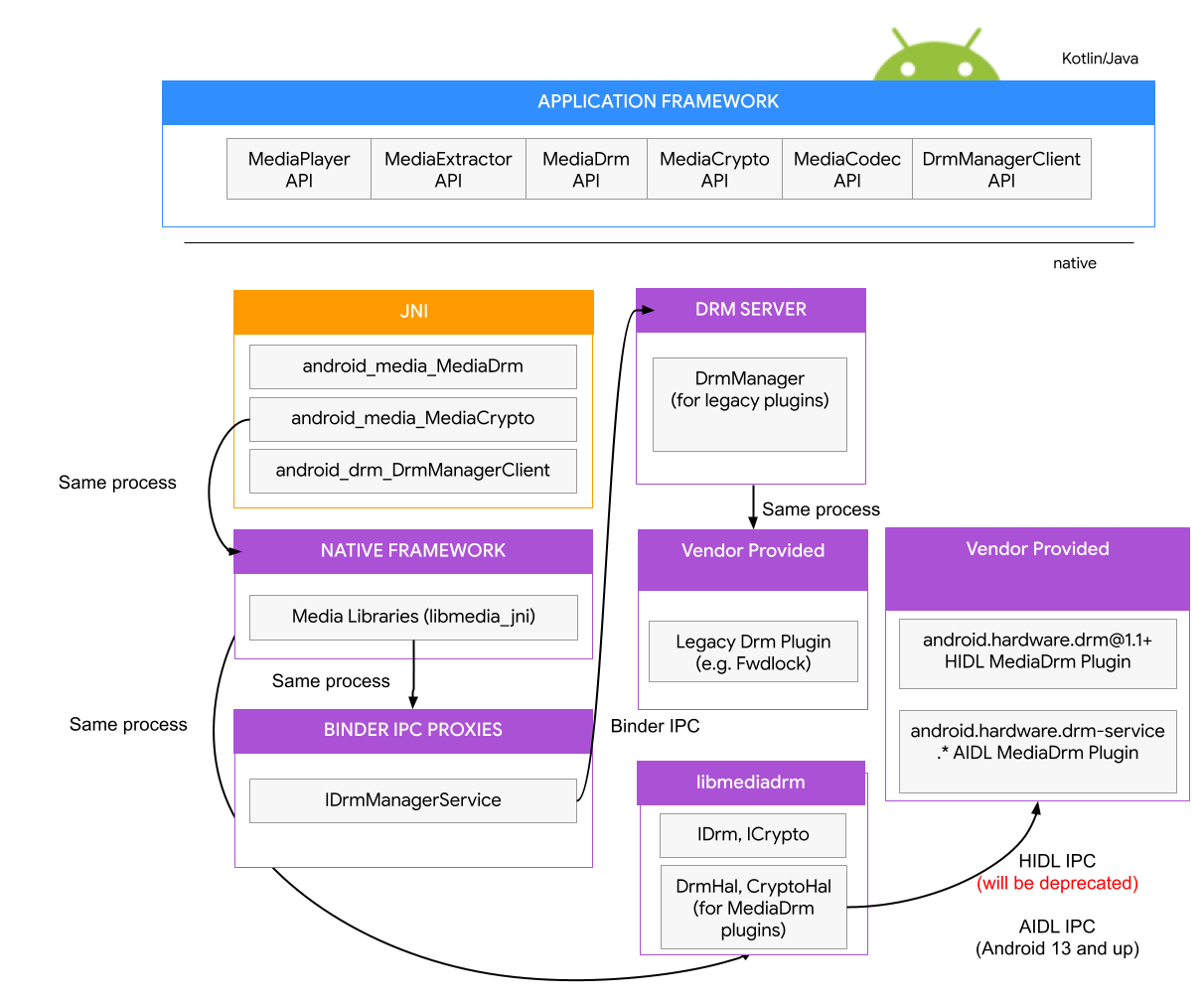
The availability of rich digital content is important to users on mobile devices. To make their content widely available, Android developers and digital content publishers need a consistent DRM implementation supported across the Android ecosystem. To make that digital content available on Android devices and to ensure that there's at least one consistent DRM available across all devices, Google provides DRM without license fees on compatible Android devices. The DRM plugin is integrated with the Android DRM framework and can use hardware-backed protection to secure premium content and user credentials.
The content protection provided by the DRM plugin depends on the security and content protection capabilities of the underlying hardware platform. The hardware capabilities of the device should include hardware secure boot to establish a chain of trust of security and protection of cryptographic keys. Content protection capabilities of the device should include protection of decrypted frames in the device and content protection through a trusted output protection mechanism. Not all hardware platforms support all of the above security and content protection features. Security is never implemented in a single place in the stack, but instead relies on the integration of hardware, software, and services. The combination of hardware security functions, a trusted boot mechanism, and an isolated secure OS for handling security functions is critical to providing a secure device.
Architecture
The DRM framework is designed to be implementation agnostic and abstracts the details of the specific DRM scheme implementation in a scheme-specific DRM plugin. The DRM framework includes simple APIs to handle complex DRM operations, acquire licenses, provision the device, associate DRM content and its license, and finally decrypt DRM content.
The Android DRM framework is implemented in two architectural layers:
- A DRM framework API, which is exposed to apps through the Android application framework.
- A native code DRM framework, which exposes an interface for DRM plugins (agents) to handle rights management and decryption for various DRM schemes.
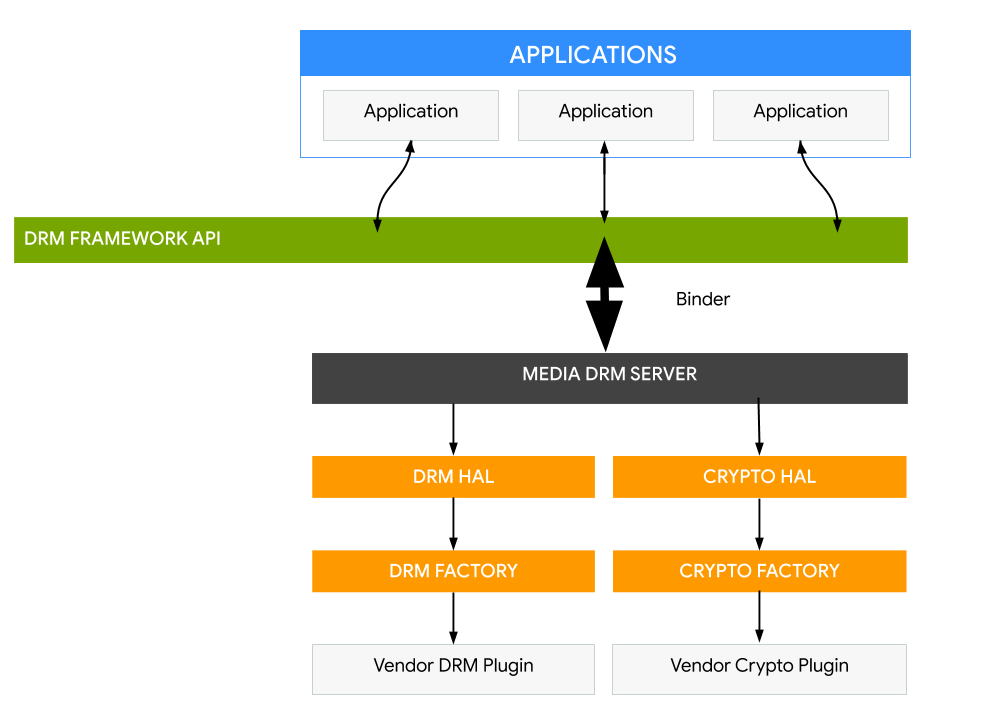
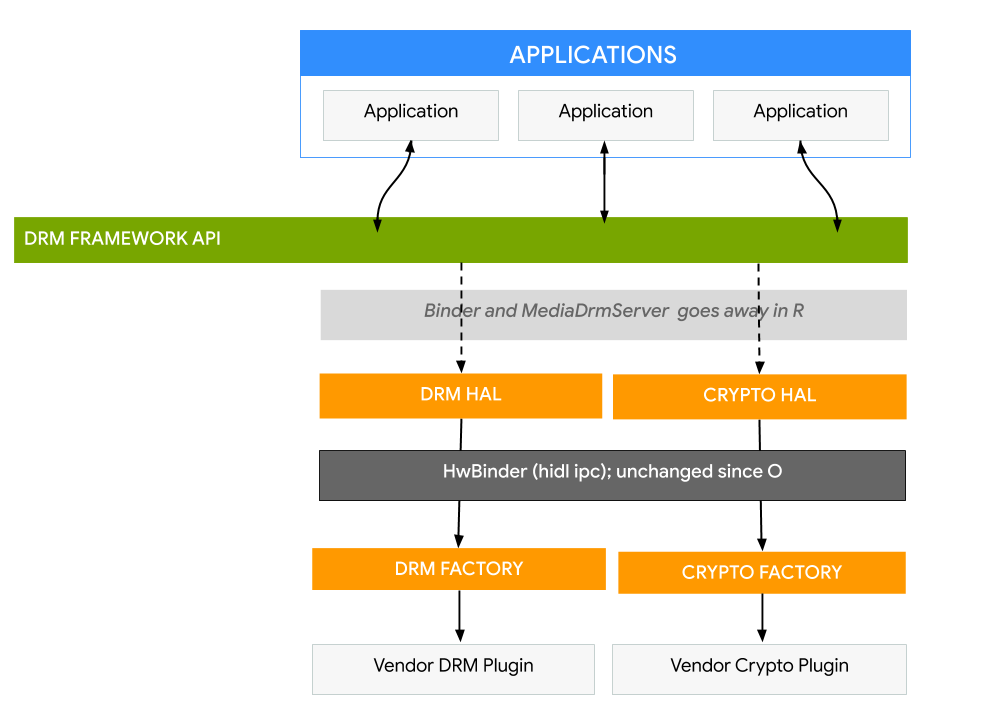
See Android Media DRM and Android Media Crypto for more details.
DRM plugins
At system startup, the DRM framework scans for HAL instances/services (described in .rc
files)
and plugins are discovered. Media DRM Server (mediadrmserver
) creates
both CryptoHal
and DrmHal
objects.
CryptoHal
and DrmHal
then call the plugins with vendor-
specific implementations.
Plugins should implement binderized HALs. Binderized HALs use the Android Interface Definition Language (AIDL), which allows the framework to be replaced without having to rebuild HALs.
Plugins are built by vendors or SOC makers and put in a /vendor
partition on
the device. All devices launching with Android 13 or higher must support binderized HALs written
in the AIDL language.
Implementation
GMS and AOSP devices release for Android 13 must use AIDL interface.
To implement new DRM frameworks APIs by a plugin:
- Add plugin service to the device’s build files.
- Update the device manifest.
- Add SELinux permissions.
- Create a
.rc
file under/vendor
. - Implement the plugin.
APIs are defined in each version of IDrmPlugin.aidl
,
ICryptoPlugin.aidl
, IDrmFactory.aidl
,
and ICryptoFactory.aidl
aidl/PLATFORM_ROOT/hardware/interfaces/drm/
Add plugin service to device build files
For example, to add AIDL interface support,
the VENDOR DEVICE/device.mk
file must include the
android.hardware.drm-service.*
packages:
PRODUCT_PACKAGES += \ android.hardware.drm-service.clearkey \ android.hardware.drm-service.widevine
Update the device manifest
The vendor manifest.xml
file for the device must include the following entries:
<hal format="aidl"> <name>android.hardware.drm</name> <version>STABLE AIDL VERSION</version> <fqname>ICryptoFactory/clearkey</fqname> <fqname>IDrmFactory/clearkey</fqname> <fqname>ICryptoFactory/widevine</fqname> <fqname>IDrmFactory/widevine</fqname> </hal>
The STABLE AIDL VERSION is the version number of each AIDL API release (e.g. 1, 2). Alternately, we recommend using vintf_fragments.
Add SELinux permissions
- Add to
VENDOR DEVICE/sepolicy/vendor/file.te
type mediadrm_vendor_data_file, file_type, data_file_type;
- Add to
VENDOR DEVICE/sepolicy/vendor/file_contexts
/vendor/bin/hw/android\.hardware\.drm-service\.clearkey u:object_r:hal_drm_clearkey_exec:s0
/data/vendor/mediadrm(/.*)? u:object_r:mediadrm_vendor_data_file:s0 - Add to
device/sepolicy/vendor/hal_drm_clearkey.te
vndbinder_use(hal_drm_clearkey) allow hal_drm_clearkey servicemanager:binder { call transfer }; allow hal_drm_clearkey hal_drm_service:service_manager add; allow hal_drm_clearkey { appdomain -isolated_app }:fd use; get_prop(ramdump, public_vendor_default_prop)
Create an RC file under /vendor
The .rc
file specifies the actions to be taken when a service is launched.
See Android Init Language for details.
Implement the plugin
- Implement the
main()
entry point inservice.cpp
of the plugin service. - Implement
ICryptoPlugin
,IDrmPlugin
,ICryptoFactory
, andIDrmFactory
. - Implement the new APIs in the plugin.
DRM plugin details
DRM plugin vendors implement DrmFactory
, CryptoFactory
, and
DRM plugin.
DrmFactory
The DrmHal
class searches for registered DRM plugin services and constructs
corresponding plugins that support a given crypto scheme through the DrmFactory
class.
IDrmFactory is the main entry point for interacting with a vendor's drm HAL through the createPlugin API. The createPlugin API is used to create IDrmPlugin instances.
::ndk::ScopedAStatus getSupportedCryptoSchemes( std::vector<::aidl::android::hardware::drm::Uuid>* _aidl_return);
getSupportedCryptoSchemes returns a list of supported crypto schemes for the AIDL drm HAL instance.
::ndk::ScopedAStatus isCryptoSchemeSupported( const ::aidl::android::hardware::drm::Uuid& in_uuid, const std::string& in_mimeType, ::aidl::android::hardware::drm::SecurityLevel in_securityLevel, bool* _aidl_return);
Determines if the plugin factory is able to construct DRM plugins that support a given crypto scheme, which is specified by a UUID.
::ndk::ScopedAStatus isContentTypeSupported(const std::string& in_mimeType, bool* _aidl_return);
Determines if the plugin factory is able to construct DRM plugins that support a
given media container format specified by mimeType
.
::ndk::ScopedAStatus createPlugin( const ::aidl::android::hardware::drm::Uuid& in_uuid, const std::string& in_appPackageName, std::shared_ptr<::aidl::android::hardware::drm::IDrmPlugin>* _aidl_return);
Constructs a DRM plugin for the crypto scheme specified by UUID.
CryptoFactory
The CryptoHal
class searches for registered DRM plugin services and constructs
corresponding plugins that support a given crypto scheme through the CryptoFactory
class.
::ndk::ScopedAStatus isCryptoSchemeSupported( const ::aidl::android::hardware::drm::Uuid& in_uuid, bool* _aidl_return);
Determines if the crypto factory is able to construct crypto plugins that support a given crypto scheme, which is specified by a UUID.
::ndk::ScopedAStatus createPlugin( const ::aidl::android::hardware::drm::Uuid& in_uuid, const std::vector<uint8_t>& in_initData, std::shared_ptr<::aidl::android::hardware::drm::ICryptoPlugin>* _aidl_return);
Determines if the plugin factory is able to construct crypto plugins that support a given crypto scheme, which is specified by a UUID.
DRM plugin APIs
The APIs are defined inhardware/interfaces/drm/aidl/aidl_api/android.hardware.drm/
VERSION/android/hardware/drm/IDrmPlugin.aidl
. The corresponding
IDrmPlugin.h
file can be found in out/Soong after the build.