Upgrade Party is a discovery flow highlighting new features for Android users whenever they receive a major OS upgrade.
A major Android operating system upgrade can be an underwhelming experience for your users. The Upgrade Party flow increases user engagement and helps users understand how to navigate their newly refreshed OS.
After upgrading to the latest OS version, users receive a push notification welcoming them to the new OS and showcasing a card flow with the new features.
To enable Upgrade Party for your Android OS, register using the Request to activate Android Upgrade Party form.
Customize the Upgrade Party experience
You can customize the Upgrade Party experience in the following ways:
- Remove irrelevant features.
- Update the language in the text blocks.
- Modify the animations, colors, and fonts to match your brand.
To customize the flow, review the guidelines in the Upgrade Party Style Guide and include your customization information in the Request to activate Android Upgrade Party form.
Upgrade Invite Style Guide
Use this style guide to modify the Upgrade Invite look to suit your own brand (screens, colors, fonts). We highly recommend keeping the sizes and the structure close to the original design.
The Upgrade Invite flow features the following three components:
- Intro screen
- Feature screen
- Outro screen
An animation on each screen helps explain each feature.
Intro screen
The intro screen contains a short animation that welcomes the user and encourages them to start the flow shown on the intro screen.
Layout
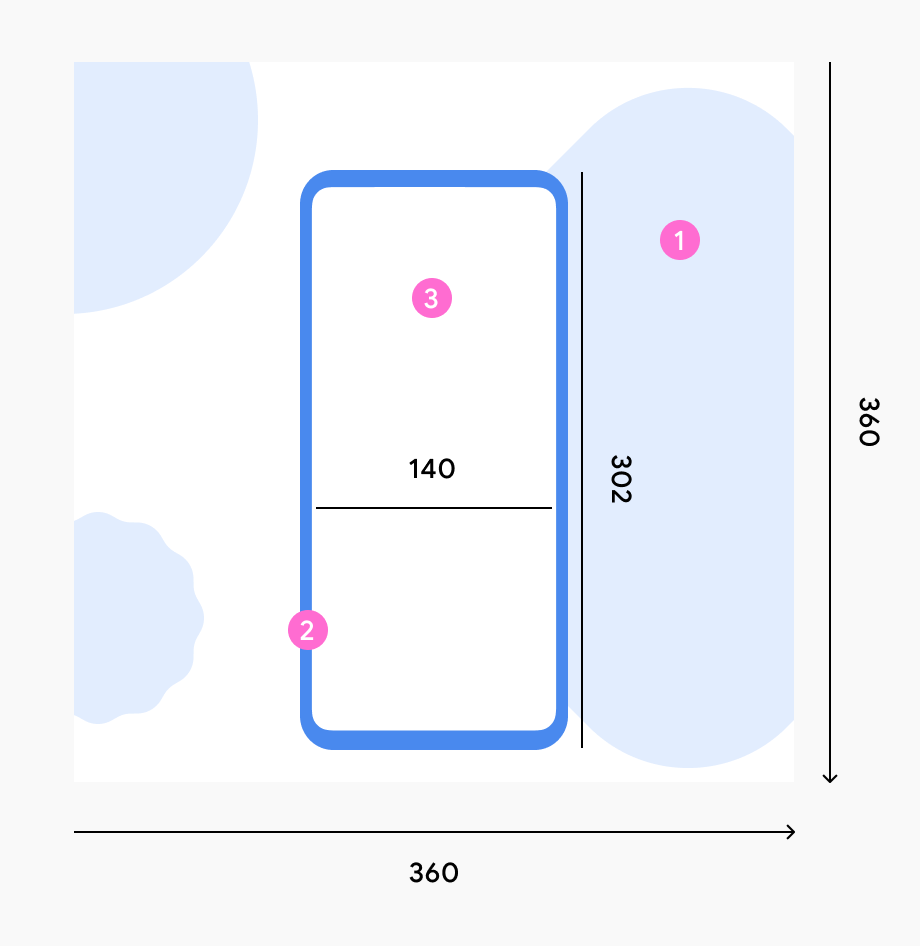
The device animation always consists of:
- White background and colorful shapes
- Device frame
- UI content
Styling
If you choose to adapt Material You styling, you can use the default colors and shapes shown in the example.
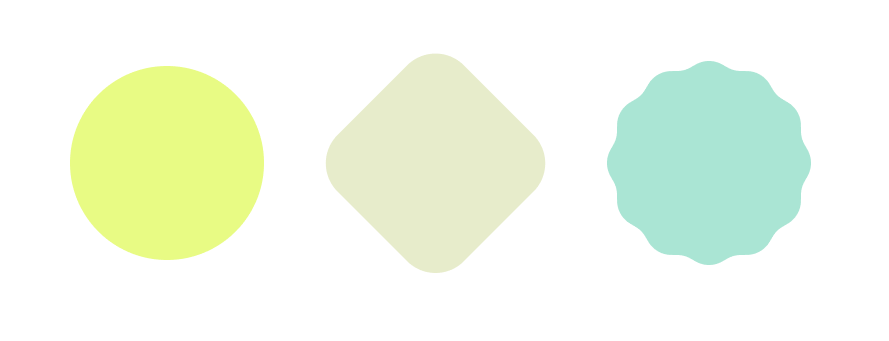
If you use your own brand color, make sure that it works well with the UI background.
Motion
Option 1: With a clock on the home screen
- Numbers enter the screen.
- Numbers move to the center while decreasing in size. The mobile phone frame appears.
- The phone decreases in size. The shapes start to enter the screen.
- The animation is completed. The shapes keep moving gently.
![]() |
![]() |
Option 2: No clock on home screen
- The mobile phone enters the screen.
- The phone moves to the center while increasing in size. The shapes start to enter the screen.
- The phone reaches the maximum size and the shapes keep moving.
![]() |
![]() |
Feature screen
Each feature screen highlights a feature in the new OS.
Layout
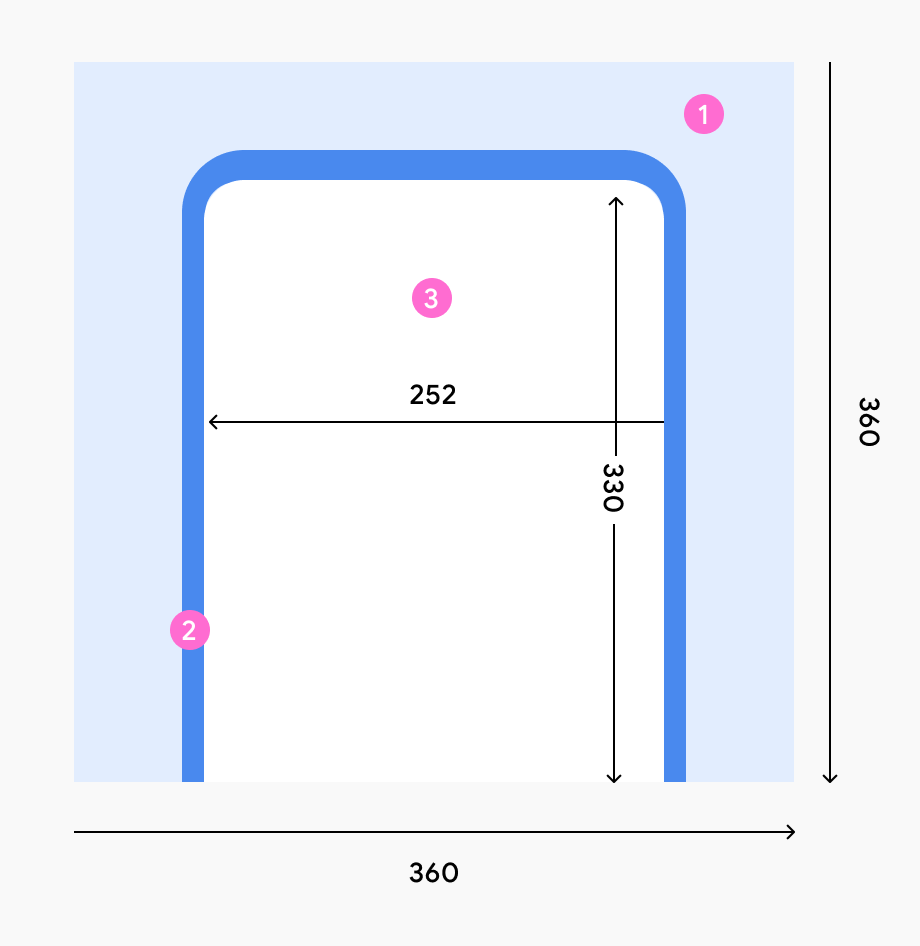
The device animation always consists of:
- Colorful background
- Device frame
- UI content
Styling
- Choose a background color that works well with the UI content and with the general appearance.
- Inside the screens, set font sizes that are readily legible. If needed, set larger font sizes.
Motion
Record an animation of each feature's flow, including touch interactions. Export the recordings as Lottie files.
Outro screen
The outro screen completes the flow. It indicates to the users that they've gone through all the screens.
Layout
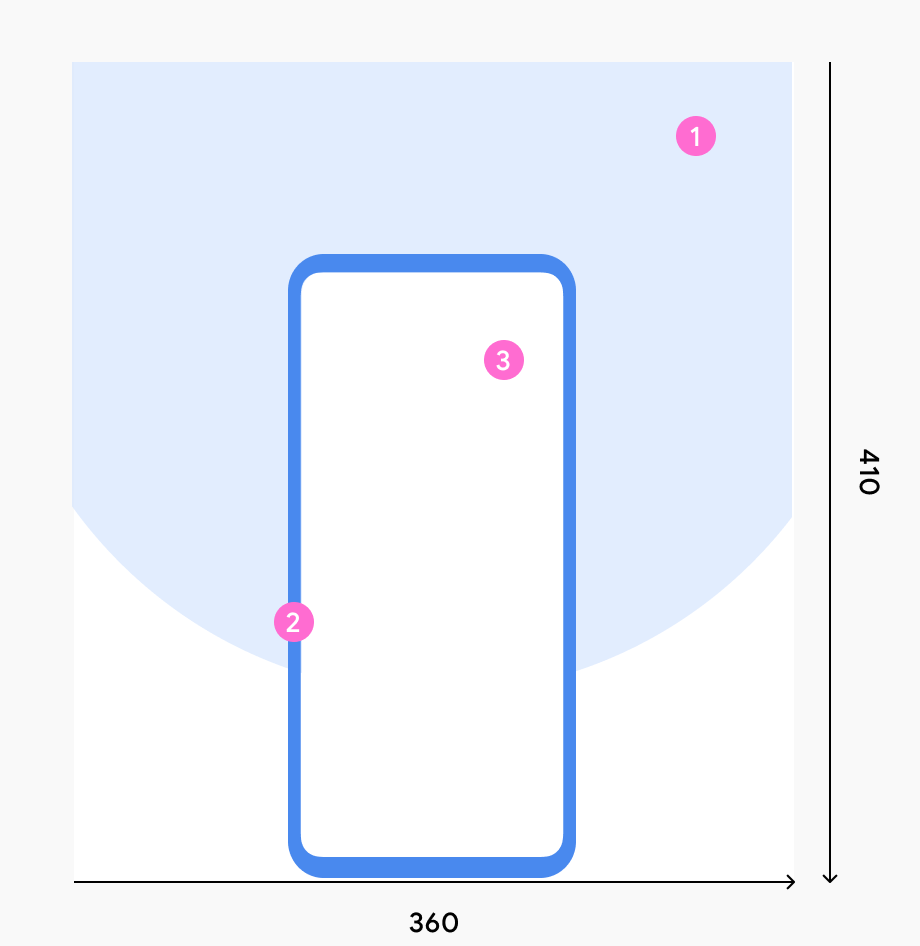
The device animation always consists of:
- Half circle on the background (plus animated shapes)
- Device frame
- UI content
Styling
- Select a background color that works well with the UI content and with the general appearance.
- Use a half-circle shape.
Motion
Follow the standard outro screen animation flow:
- The mobile phone moves up a bit.
- A circle appears in the background.
- Shapes come up from behind the mobile phone.
- The shapes fly out of the background like confetti.
![]() |
![]() |
Trigger the Upgrade Party with the SDK
By default, the Android OS sends users a push notification showcasing a card flow with the latest features as soon as they upgrade. If you prefer to trigger the flow from your own push notification or an app, use the Android SDK.
Prerequisites
Make sure that your app's build file uses the following values:
minSdkVersion
of 14 or highercompileSdkVersion
of 28 or higher
Configure your app
- Extract the library from the provided Zip file, and place it in your repository.
Add the dependencies for the Google Growth SDK to your module's app-level Gradle file, normally
app/build.gradle
:dependencies { implementation files('<PATH_TO_BINARY>/play-services-growth-16.1.0-eap04.aar') }
Include the following libraries as dependencies:
com.google.android.gms:play-services-base:18.0.1 com.google.android.gms:play-services-basement:18.0.0 com.google.android.gms:play-services-tasks:18.0.1
Use the API
To initialize a client, use the
UpgradeParty
class and set theactivity
parameter.import com.google.android.gms.growth.UpgradeParty; UpgradeParty.getClient(activity);
The resulting UpgradePartyClient
exposes the API functionality.
isEligibleForUpgradeParty
Task<Boolean> isEligibleForUpgradeParty();
Returns a Task
object that asynchronously verifies if the user is eligible for an
Upgrade Party. The resulting boolean value indicates whether the user is
eligible or not.
invokeUpgradeParty
Task<Void> invokeUpgradeParty();
Invokes an Upgrade Party Activity
. The resulting Task
object indicates
whether an error occurred when starting the activity.
Errors
Both API methods can fail with an exception of type
com.google.android.gms.common.api.ApiException
. In such cases, the caller is
expected to retry at a later time.
Expected common status codes include:
INTERNAL_ERROR
: Represents any error in the flow of the underlying logic.TIMEOUT
: Shown when the request couldn't be handled in a timely manner.API_NOT_CONNECTED
: Means the API isn't available (for example, theUpgradeParty
module is not ready yet).DEVELOPER_ERROR
: Shown when the calling package isn't allowed to access theUpgradeParty
class.
SDK example
See the following example of an app that invokes the SDK:
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import com.google.android.gms.growth.UpgradeParty;
import com.google.android.gms.growth.UpgradePartyClient;
public class SampleActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
final UpgradePartyClient client = UpgradeParty.getClient(this);
// Hide the invocation button initially
View startUpgradePartyButton = findViewById(R.id.__some_button__);
startUpgradePartyButton.setVisibility(View.GONE);
// Register an onClick handler to invoke the Upgrade Party Activity
startUpgradePartyButton
.setOnClickListener(
view -> {
client
.invokeUpgradeParty()
.addOnCompleteListener(
task -> {
if (!task.isSuccessful()) {
// Do something with error, see task.getException()
}
});
});
}
// Check if eligible for an Upgrade Party
client
.isEligibleForUpgradeParty()
.addOnCompleteListener(
task -> {
if (!task.isSuccessful()) {
// Do something with error, see task.getException()
} else {
// Show/Hide invocation button, based on the result
int visibility =
task.getResult() ? View.VISIBLE : View.GONE;
startUpgradePartyButton..setVisibility(visibility);
}
});
}
Test the Upgrade Party flow
Follow these steps to test the Upgrade Party flow your users see when they upgrade the Android OS:
- On the test Android device, go to Settings > Google > Upgrade Party debug.
- Select Report Upgrade and then Open Upgrade Party. This starts the relevant flow, based on the device OS version. For example, the Android 12 Upgrade Party plays on a device running the Android 12 OS.