Text classifier uses machine learning techniques to help developers classify text.
Android 11 release text classifier
Android 11 introduces an updatable default
implementation of the text classifier service in the
ExtServices module. On devices running Android 11
or higher, the
getTextClassifier()
method returns this default implementation in the ExtServices module.
Device manufacturers are recommended to use this implementation of
TextClassifierService
as it can be updated through Mainline OTA
updates.
Android 11 also removes the local text classifier
default implementation previously introduced in
Android 8.1. As a result,
getLocalTextClassifier()
returns a NO_OP
text classifier. Instead of the local
implementation, you should use the
getDefaultTextClassifierImplementation()
method.
For device manufacturers who might want to use their own text classifying
algorithms, they can implement a custom text classifier service by specifying
config_defaultTextClassifierPackage
in the config.xml
file. If this configuration isn't specified, the default system implementation
is used. Custom implementations can get an instance of the default
implementation by calling
TextClassifierService.getDefaultTextClassifierImplementation(Context)
.
For more information, see
Implementing a custom text classifier service.
Testing
To validate your implementation of the text classifier service, use the
Compatibility Test Suite (CTS) tests in
platform/cts/tests/tests/textclassifier/
.
Android 10 release text classifier enhancements
Android 10 introduces two methods to the
TextClassifier API:
suggestConversationActions
and
detectLanguage
.
The suggestConversationActions
method generates suggested replies
and actions from a given conversation and the detectLanguage
method
detects the language of the text.
The model files for these methods are shown below and can be found in
external/libtextclassifier/models/
.
suggestionConversationActions
:actions_suggestions.universal.model
detectLanguage
:lang_id.model
To release a device with the latest model files in your factory image, do the following:
Fetch the latest model files.
external/libtextclassifier/models/update.sh
- Rename the downloaded files to replace the existing ones.
- Verify the setup.
adb shell dumpsys textclassification
This is a sample of the output of that command.
TextClassifierImpl: Annotator model file(s): ModelFile { path=/etc/textclassifier/textclassifier.universal.model name=textclassifier.universal.model version=608 locales=und } ModelFile { path=/etc/textclassifier/textclassifier.en.model name=textclassifier.en.model version=608 locales=en } LangID model file(s): ModelFile { path=/etc/textclassifier/lang_id.model name=lang_id.model version=0 locales=und } Actions model file(s): ModelFile { path=/etc/textclassifier/actions_suggestions.universal.model name=actions_suggestions.universal.model version=0 locales=und }
Android 9 release text classifier enhancements
Android 9 extended the text classification framework introduced in Android 8.1 with the new text classifier service. The text classifier service is the recommended way for OEMs to provide text classification system support. The text classifier service can be part of any system APK and can be updated when necessary.
Android 9 includes a default text classifier
service implementation
(
TextClassifierImpl
) that is used unless you replace it with a
custom text classifier service
implementation.
Implement a custom text classifier service
The following sections describe how to implement a custom text classifier service that you develop.
Extend android.service.textclassifier.TextClassifierService
public final class TextClassifierServiceImpl extends TextClassifierService { // Returns TextClassifierImpl. private final TextClassifier tc = getLocalTextClassifier(); @Override public void onSuggestSelection( @Nullable TextClassificationSessionId sessionId, @NonNull TextSelection.Request request, @NonNull CancellationSignal cancellationSignal, @NonNull Callback<TextSelection> callback) { CompletableFuture.supplyAsync( () -> tc.suggestSelection(request)) .thenAccept(r -> callback.onSuccess(r)); } @Override public void onClassifyText( @Nullable TextClassificationSessionId sessionId, @NonNull TextClassification.Request request, @NonNull CancellationSignal cancellationSignal, @NonNull Callback<TextClassification> callback) { ... } @Override public void onGenerateLinks( @Nullable TextClassificationSessionId sessionId, @NonNull TextLinks.Request request, @NonNull CancellationSignal cancellationSignal, @NonNull Callback<TextLinks> callback) { ... } ... }
Define the service in the Android manifest
[AndroidManifest.xml]
<service android:name=".TextClassifierServiceImpl" android:permission="android.permission.BIND_TEXTCLASSIFIER_SERVICE"> <intent-filter> <action android:name= "android.service.textclassifier.TextClassifierService"/> </intent-filter> </service>
Note that the service must require the
android.permission.BIND_TEXTCLASSIFIER_SERVICE
permission and must
specify the
android.service.textclassifier.TextClassifierService
intent
action.
Set a system default text classifier service in the config overlay
[config.xml]
<string name="config_defaultTextClassifierPackage" translatable="false">com.example.textclassifierservice</string>
Build the text classifier service into the system image
Your custom text classifier service can be a standalone APK that is built
into the system image or a part of another system APK. The system uses
PackageManager.MATCH_SYSTEM_ONLY
to resolve the service.
Testing
Run tests in android.view.textclassifier.cts
.
Other text classification changes in Android 9
Refer to Inspecting installed language modules.
Android 9 model files are incompatible with Android 8.x model files.
Android 9 model files have the naming pattern:
texclassifier.[language-code].model
(for example,
textclassifier.en.model
)
instead of textclassifier.smartselection.en.model
in Android 8.x.
Obtain the latest text classification model files
To obtain the most up-to-date models the following script can be run, which updates the TextClassifier models in the source tree:
external/libtextclassifier/native/models/update.sh
Android release 8.1 text classifier
Android 8.1 introduced the TextClassfier API to implement text classification.
TextClassificationManager tcm = context.getSystemService(TextClassificationManager.class); TextClassifier classifier = tcm.getTextClassifier(); TextSelection selection = classifier.suggestSelection(...); TextClassification classification = classifier.classifyText(...);
Developers can set a custom text classifier:
tcm.setTextClassifier(customTextClassifier);
But if an app developer sets the text classifier to null
, a system
default text classifier is returned for getTextClassifier()
.
See android.view.textclassifier.TextClassifierImpl
.
TextView and WebView use TextClassifier for smart selection and smart text share features.
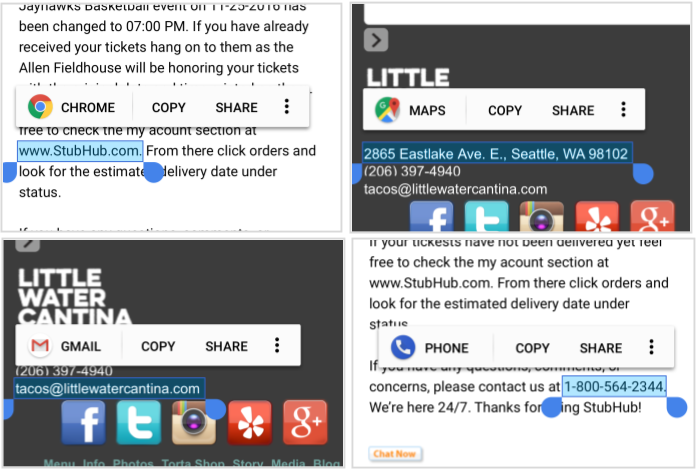
Figure 1. TextClassifier usage
TextClassifier neural-net models
The Android Open Source Project (AOSP) features a number of neural network models for classifying text. Each model file is trained for a single language. You can install any combination of models. The models are defined in:
external/libtextclassifier/Android.mk
Preinstall language models on devices
You can specify a bundle of language models and install them on a device:
# ----------------------- # Smart Selection bundles # ----------------------- include $(CLEAR_VARS) LOCAL_MODULE := textclassifier.smartselection.bundle1 LOCAL_REQUIRED_MODULES := textclassifier.smartselection.en.model LOCAL_REQUIRED_MODULES += textclassifier.smartselection.es.model LOCAL_REQUIRED_MODULES += textclassifier.smartselection.de.model LOCAL_REQUIRED_MODULES += textclassifier.smartselection.fr.model include $(BUILD_STATIC_LIBRARY)
For example, in device/google/marlin/device-common.mk
.
# TextClassifier smart selection model files
PRODUCT_PACKAGES += \
textclassifier.smartselection.bundle1
Inspect installed language modules
Use ADB to list the files in the directory:
$ adb shell ls -l /etc/textclassifier -rw-r--r-- 1 root root ... textclassifier.smartselection.de.model -rw-r--r-- 1 root root ... textclassifier.smartselection.en.model -rw-r--r-- 1 root root ... textclassifier.smartselection.es.model -rw-r--r-- 1 root root ... textclassifier.smartselection.fr.model
Model updates
Models can be updated either by having a new model included as part of a system
image update, or dynamically by having a system component that triggers
an update through the system API ACTION_UPDATE_SMART_SELECTION
intent. By broadcasting this system API intent, the framework is able to
update the language model of the currently set language. The models themselves
contain the supported language and a version number so the latest appropriate
model is used.
So you don't need to preload models for all languages because they can be added later. If no model file is found for the specified language, text classification returns no-op values.
Compatibility Test Suite tests
The associated Android Compatibility Test Suite (CTS) tests can be found in:
cts/tests/tests/view/src/android/view/textclassifier/cts/TextClassificationManagerTest.java
cts/tests/tests/widget/src/android/widget/cts/TextViewTest.java
testSmartSelection
testSmartSelection_dragSelection
testSmartSelection_resetSelection